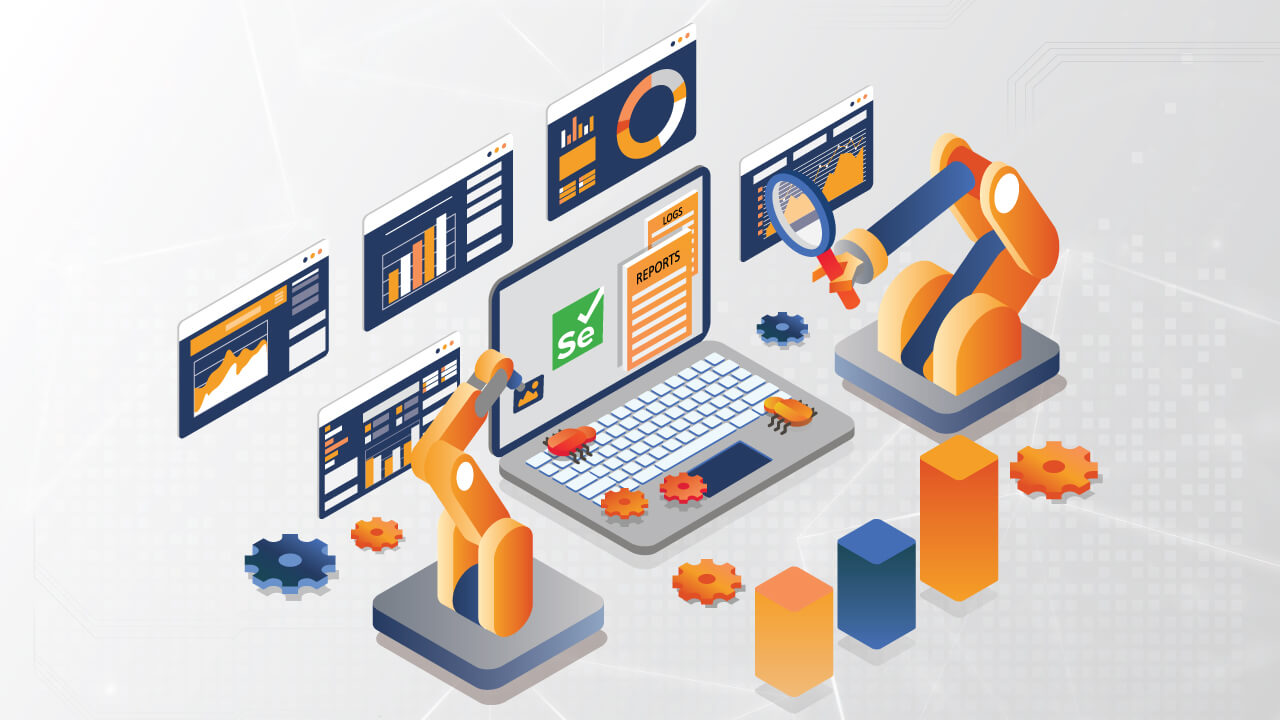
Selenium is the most famous open-source test automation testing framework. It’s open-source and widely used. Its importance in web testing can’t be overstated. Selenium allows testers to automate browsers. This helps in finding bugs early and improving software quality. But basic tests aren’t always enough. Advanced techniques can make a huge difference.
This blog on what is Selenium explores its advanced techniques. We’ll cover data-driven testing, the Page Object Model, and cross-browser testing. These methods enhance testing efficiency and accuracy. Let’s explore these techniques.
Understanding Advanced Web Testing
Advanced web testing goes beyond the basics. It involves more complex scenarios. This type of testing ensures that web applications work under different conditions. It tests for edge cases and unusual user behaviors. Advanced testing is essential for high-quality software.
Advanced web testing has many challenges. Handling dynamic content is tough. Different browsers behave differently. There are also performance issues. Writing and maintaining complex tests can be difficult. But overcoming these challenges is crucial. It leads to more robust and reliable web applications.
Data-Driven Testing
Data-driven testing is a powerful technique. It uses external data to drive test cases. Instead of hardcoding test data, you use external files. These can be spreadsheets, databases, or CSV files. This method makes tests more flexible and reusable.
Using data-driven testing in Selenium has many benefits. It allows for easy test case variations. You can test multiple scenarios with the same script. This saves time and effort. It also makes it easier to maintain tests.
Simple example of data driven testing in Selenium:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class DataDrivenTest {
WebDriver driver;
@Test(dataProvider = “loginData”)
public void loginTest(String username, String password) {
System.setProperty(“webdriver.chrome.driver”, “path/to/chromedriver”);
driver = new ChromeDriver();
driver.get(“https://example.com/login”);
driver.findElement(By.id(“username”)).sendKeys(username);
driver.findElement(By.id(“password”)).sendKeys(password);
driver.findElement(By.id(“loginButton”)).click();
driver.quit();
}
@DataProvider(name = “loginData”)
public Object[][] getData() {
return new Object[][] {
{“user1”, “pass1”},
{“user2”, “pass2”},
{“user3”, “pass3”}
};
}
}
In this example, the DataProvider supplies login data. The loginTest method uses this data to test different login scenarios. This way, the same test script can handle multiple data sets. It’s efficient and easy to manage.
Page Object Model (POM)
The Page Object Model (POM) is a design pattern. It makes test automation more efficient. POM helps organize test code better. It separates the test logic from the page structure. This makes tests easier to maintain and read.
Using POM, you create a separate class for each web page. This class contains methods to interact with the page. These methods are then used in test scripts. If the web page changes, you only update the page class. The test scripts remain unchanged.
Here’s how to implement POM in Selenium:
- Create a Page Class:
java
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver;
public class LoginPage { WebDriver driver;
By username = By.id(“username”);
By password = By.id(“password”);
By loginButton = By.id(“loginButton”);
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void setUsername(String strUsername) {
driver.findElement(username).sendKeys(strUsername);
}
public void setPassword(String strPassword) {
driver.findElement(password).sendKeys(strPassword);
}
public void clickLogin() {
driver.findElement(loginButton).click();
}
}
2. Use the Page Class in Tests:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.Test;
public class LoginTest {
WebDriver driver;
@Test
public void testLogin() {
System.setProperty(“webdriver.chrome.driver”, “path/to/chromedriver”);
driver = new ChromeDriver();
driver.get(“https://example.com/login”);
LoginPage loginPage = new LoginPage(driver);
loginPage.setUsername(“user1”);
loginPage.setPassword(“pass1”);
loginPage.clickLogin();
driver.quit();
}
}
In this example, the LoginPage class represents the login page. It contains methods to interact with the page. The LoginTest class uses these methods to perform the login test. This makes the code clean and easy to manage.
Cross-Browser Testing
Cross-browser testing is essential. Different browsers can render web pages differently. A web application must work correctly across all browsers. This ensures a consistent user experience.
Selenium supports cross-browser testing. You can run tests on different browsers using the same script. This is efficient and saves time. Here’s how to perform cross-browser testing in Selenium:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.annotations.Parameters;
import org.testng.annotations.Test;
public class CrossBrowserTest {
WebDriver driver;
@Test
@Parameters(“browser”)
public void testLogin(String browser) {
if (browser.equalsIgnoreCase(“chrome”)) {
System.setProperty(“webdriver.chrome.driver”, “path/to/chromedriver”);
driver = new ChromeDriver();
} else if (browser.equalsIgnoreCase(“firefox”)) {
System.setProperty(“webdriver.gecko.driver”, “path/to/geckodriver”);
driver = new FirefoxDriver();
}
driver.get(“https://example.com/login”);
// Perform login test steps
driver.quit();
}
}
In this example, the testLogin method takes a browser parameter. Based on this parameter, it initializes the corresponding browser driver. This way, the same test script can run on different browsers.
Integrating Selenium with tools such as LambdaTest allows cross-browser testing much more effectively, mainly because LambdaTest is in the cloud. It will enable you to test on any browser over any OS; this ensures full coverage in cross-browser testing.
Using LambdaTest with Selenium is straightforward. You can run tests on LambdaTest’s cloud infrastructure. This eliminates the need to maintain a local test environment. Here’s a simple example:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.MalformedURLException;
import java.net.URL;
public class LambdaTestTrial {
public static void main(String[] args) throws MalformedURLException {
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(“browserName”, “chrome”);
capabilities.setCapability(“platform”, “Windows 11”);
capabilities.setCapability(“version”, “102”);
WebDriver driver = new RemoteWebDriver(new URL(“https://username:accesskey@hub.lambdatest.com/wd/hub”), capabilities);
driver.get(“https://example.com”);
// Perform test steps
driver.quit();
}
}
In this example, the RemoteWebDriver connects to LambdaTest’s cloud. The DesiredCapabilities class specifies the browser and platform. This allows you to run tests on different browser and OS combinations easily.
Handling Dynamic Web Elements
Dynamic web elements can be tricky. They change or load at different times. This creates challenges in automated testing. The elements may not be present when the script runs. Locating and interacting with these elements becomes difficult.
But don’t worry, there are strategies to handle this. First, use explicit waits. This tells Selenium to wait until a condition is met. For example, waiting for an element to be clickable:
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(By.id(“dynamicElement”)));
element.click();
Another strategy is using CSS selectors and XPath. These can locate elements based on complex criteria. For example, finding an element by partial text:
WebElement element = driver.findElement(By.xpath(“//*[contains(text(), ‘partialText’)]”));
element.click();
Practical example: Handling a dynamic dropdown menu. Wait for the dropdown to be visible, then select an option:
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement dropdown = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id(“dropdownMenu”)));
dropdown.click();
WebElement option = driver.findElement(By.xpath(“//option[text()=’OptionText’]”));
option.click();
Best practices: Always use explicit waits for dynamic elements. Avoid using Thread.sleep as it’s not reliable. Use robust selectors like CSS and XPath. Test different scenarios to ensure reliability.
Parallel Test Execution
Parallel test execution runs multiple tests simultaneously. This speeds up the testing process. It’s especially useful for large test suites.
Benefits are clear. Faster feedback, better resource utilization, and reduced testing time. To set this up in Selenium, use TestNG or JUnit. Both support parallel execution.
Setting up parallel tests with TestNG:
- Create a TestNG XML file:
<!DOCTYPE suite SYSTEM “http://testng.org/testng-1.0.dtd”>
<suite name=”Suite” parallel=”tests” thread-count=”2″>
<test name=”Test1″>
<classes>
<class name=”TestPackage.TestClass1″/>
</classes>
</test>
<test name=”Test2″>
<classes>
<class name=”TestPackage.TestClass2″/>
</classes>
</test>
</suite>
- Configure tests to run in parallel. Set the parallel attribute to tests and specify thread-count.
Tools and frameworks: Besides TestNG, there’s JUnit and Selenium Grid. Selenium Grid is powerful for parallel tests across different machines.
Setting up Selenium Grid:
Start the hub:
java -jar selenium-server-standalone.jar -role hub
Start the nodes:
java -jar selenium-server-standalone.jar -role node -hub http://localhost:4444/grid/register
Run tests across different browsers and machines. This maximizes efficiency and coverage.
Integrating LambdaTest for Enhanced Testing
LambdaTest is a cloud-based platform. It supports cross-browser and parallel testing. Integrating LambdaTest with Selenium brings many benefits. It provides access to numerous browsers and OS combinations. This ensures comprehensive test coverage.
- Step-by-step guide to setting up LambdaTest:
Add LambdaTest dependencies to your project:
<dependency>
<groupId>com.lambdatest</groupId>
<artifactId>lambdatest</artifactId>
<version>1.0.0</version>
</dependency>
- Set up your test to run on LambdaTest:
java import org.openqa.selenium.WebDriver; import org.openqa.selenium.remote.DesiredCapabilities; import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.MalformedURLException; import java.net.URL;
public class LambdaTestTrial { public static void main(String[] args) throws MalformedURLException { DesiredCapabilities capabilities = new DesiredCapabilities(); capabilities.setCapability(“browserName”, “chrome”); capabilities.setCapability(“version”, “latest”); capabilities.setCapability(“platform”, “Windows 10”);
WebDriver driver = new RemoteWebDriver(new URL(“https://username:accesskey@hub.lambdatest.com/wd/hub”), capabilities);
driver.get(“https://example.com”);
// Perform test steps
driver.quit();
}
}
Real-world examples: Use LambdaTest for cross-browser testing. It handles different browser and OS combinations effortlessly. Use it for parallel testing. It runs multiple tests at the same time, saving time and resources. Overcoming challenges is easier with LambdaTest. It ensures your web application works seamlessly across all platforms.
Advanced Reporting
Detailed test reports are crucial. They help in understanding test results better. Advanced reporting provides insights into test execution. It highlights issues and helps in debugging.
Generating advanced reports in Selenium is possible with TestNG or JUnit. Integrate with reporting tools like Allure or ExtentReports.
Using ExtentReports:
1. Add ExtentReports dependency:
xml
<dependency>
<groupId>com.aventstack</groupId>
<artifactId>extentreports</artifactId>
<version>4.0.9</version>
</dependency>
2. Set up ExtentReports in your test:
import com.aventstack.extentreports.reporter.ExtentHtmlReporter;
import com.aventstack.extentreports.ExtentReports;
import com.aventstack.extentreports.ExtentTest;
public class ReportExample {
public static void main(String[] args) {
ExtentHtmlReporter htmlReporter = new ExtentHtmlReporter(“extent.html”);
ExtentReports extent = new ExtentReports();
extent.attachReporter(htmlReporter);
ExtentTest test = extent.createTest(“MyFirstTest”, “Sample description”);
test.pass(“Test passed”);
extent.flush();
}
}
Integrate with reporting tools for detailed analysis. Allure provides a rich interface for test reports. It integrates seamlessly with Selenium and other testing frameworks.
Using Allure with TestNG:
1. Add Allure TestNG dependency:
xml
<dependency>
<groupId>io.qameta.allure</groupId>
<artifactId>allure-testng</artifactId>
<version>2.13.8</version>
</dependency>
2. Annotate your test methods:
import io.qameta.allure.Step; import org.testng.annotations.Test;
public class AllureExample {
@Test
@Step(“Sample test step”)
public void sampleTest() {
// Test steps
}
}
Generate Allure reports using command line:
shell
allure serve target/allure-results
Advanced reporting provides clear insights. It helps in identifying issues quickly. Detailed reports are essential for effective test analysis.
Conclusion
We’ve explored advanced Selenium techniques. Handling dynamic web elements, parallel test execution, and cross-browser testing are vital. Integrating LambdaTest enhances testing efficiency. Advanced reporting tools provide detailed insights.
Implement these techniques for more effective web testing. Stay updated with the latest trends and tools in Selenium. Continuous learning and adaptation are key to successful web testing. Happy testing!